SQL is a programming language that is widely used for managing and querying databases. It is a powerful tool that can be used to retrieve, manipulate, and analyze data stored in databases. SQL stands for Structured Query Language, and it is designed to work with relational databases, which are databases that store data in tables.
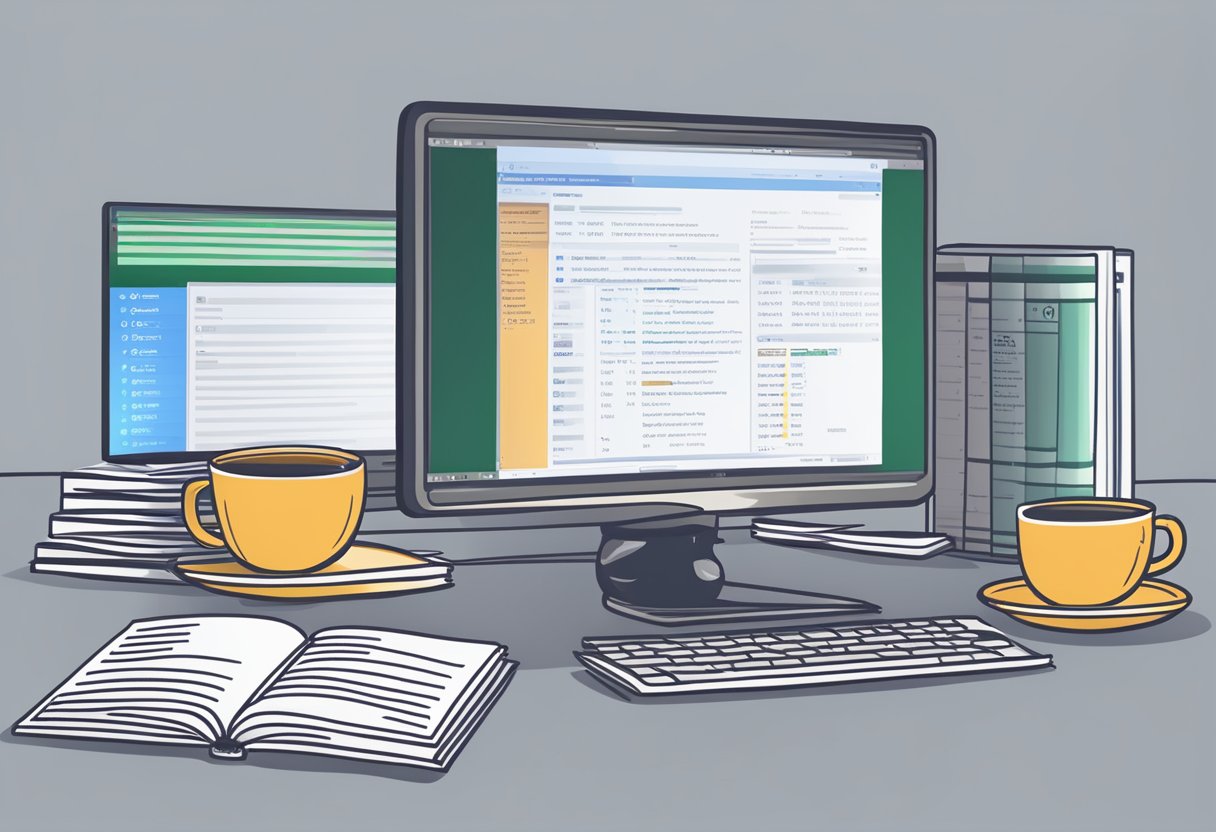
Database management is an important task for any organization that deals with large amounts of data. A database is a collection of related data that is organized in a structured way. Databases are used to store, retrieve, and manage data efficiently. Database management involves tasks such as designing, creating, and maintaining databases, as well as performing backups and ensuring data security. SQL is an essential tool for database management, as it allows users to interact with databases in a powerful and flexible way.
Learning SQL basics is an important step for anyone who wants to work with databases. SQL beginner courses cover topics such as creating tables, inserting data, querying data, and modifying data. By learning SQL, users can gain a better understanding of how databases work, and they can become more effective at managing and analyzing data. Whether you are a data analyst, a database administrator, or a software developer, SQL is a valuable skill to have.
Understanding the Basics of SQL
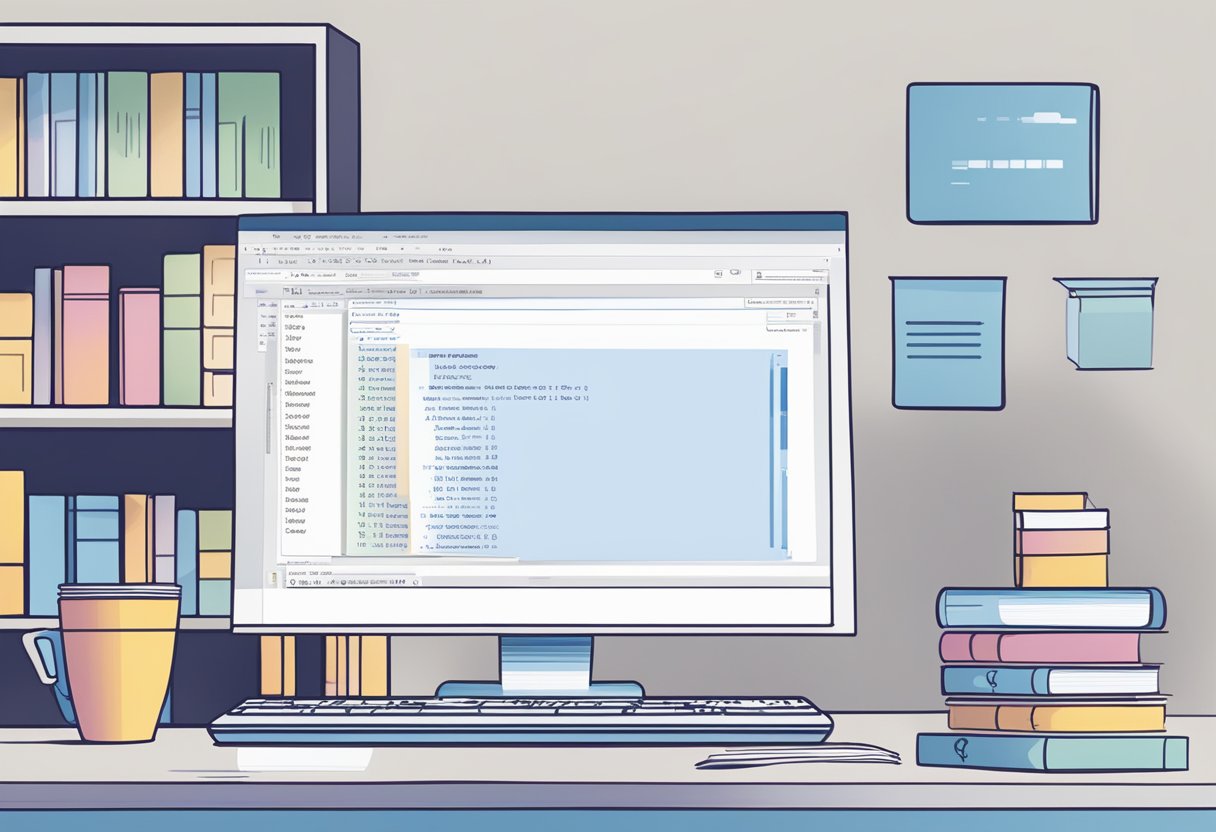
What Is SQL and Its Role in Database Management
SQL, or Structured Query Language, is a programming language used to manage and manipulate relational databases. It is widely used by developers, data analysts, and database administrators to perform various tasks such as creating, modifying, and querying databases. SQL is a standard language used by most relational database management systems (RDBMS) such as MySQL, PostgreSQL, and Oracle.
SQL plays a crucial role in database management as it allows users to interact with the database and retrieve data as per their requirements. It is used to create and modify database structures, insert, update, and delete data, and perform various operations such as sorting, filtering, and grouping of data.
SQL Syntax: Keywords and Commands
SQL syntax consists of various keywords and commands that are used to perform different operations on databases. Some of the commonly used SQL commands are SELECT, INSERT, UPDATE, DELETE, CREATE, ALTER, and DROP. These commands are used to perform operations such as retrieving data from tables, inserting new records, updating existing records, deleting records, creating new tables, modifying existing tables, and dropping tables.
SQL keywords are used to specify the type of operation that needs to be performed. Some of the commonly used SQL keywords are SELECT, FROM, WHERE, GROUP BY, HAVING, and ORDER BY. These keywords are used to specify the columns to be selected, the table from which data needs to be retrieved, the conditions to be applied to the data, and the order in which data needs to be sorted.
Data Types and Databases
SQL supports various data types such as INTEGER, FLOAT, VARCHAR, DATE, and BOOLEAN. These data types are used to specify the type of data that can be stored in a column of a table. For example, INTEGER is used to store numeric data, VARCHAR is used to store character strings, and DATE is used to store date values.
SQL is used to manage both relational and non-relational databases. Relational databases are based on the relational model and consist of tables that are related to each other through keys. Non-relational databases, on the other hand, do not follow the relational model and are used to store unstructured data such as images, videos, and documents.
In conclusion, understanding the basics of SQL is essential for anyone working with databases. SQL is a powerful language that allows users to manage and manipulate databases efficiently. By mastering SQL syntax, keywords, and data types, users can perform various operations on databases and retrieve data as per their requirements.
Setting Up the Database Environment
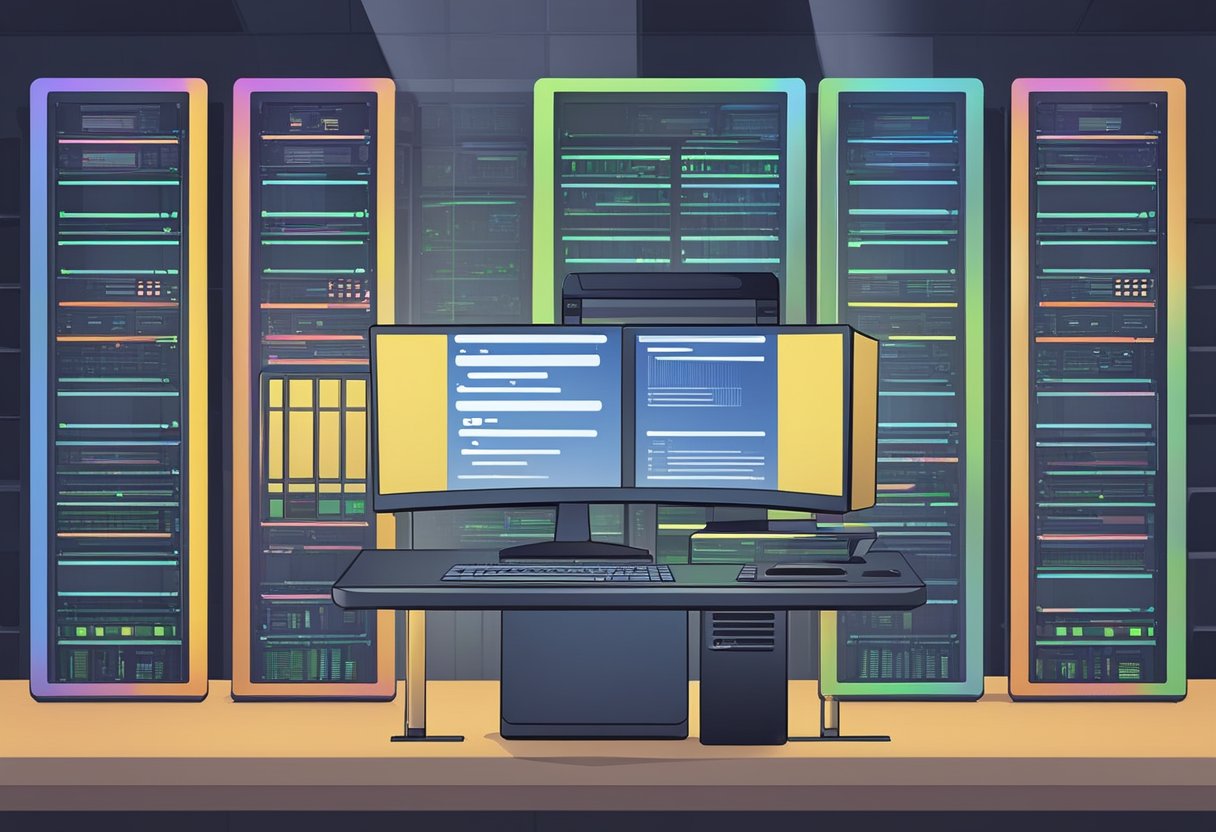
Choosing the Right SQL Database
When it comes to choosing the right SQL database, there are several options available. Some of the most popular ones are SQL Server, MySQL, PostgreSQL, and SQLite. The choice of database depends on the specific requirements of the project. SQL Server is a popular choice for enterprise-level applications, while MySQL and PostgreSQL are commonly used for web applications. SQLite is a lightweight database that is ideal for mobile and desktop applications.
Installing SQL Server, MySQL, PostgreSQL, or SQLite
Once you have chosen the database that best suits your needs, the next step is to install it. The installation process varies depending on the database you choose. For SQL Server, you can download the installer from the Microsoft website and follow the installation wizard. MySQL and PostgreSQL can be installed using package managers or downloaded as standalone installers. SQLite can be used as a library within an application or installed as a standalone executable.
Creating Your First Database and Table
After installing the database, the next step is to create a database and table. In SQL Server, you can use SQL Server Management Studio (SSMS) to create a new database and table. In MySQL, you can use the MySQL Workbench or command-line interface to create a database and table. In PostgreSQL, you can use the pgAdmin tool or command-line interface to create a database and table. In SQLite, you can use the command-line interface or a GUI tool like DB Browser for SQLite to create a database and table.
To create a database, you need to define the schema, which includes the tables, columns, and relationships between the tables. You can use SQL statements to create the schema. For example, to create a table in SQL Server, you can use the following statement:
CREATE TABLE Customers (
CustomerID int PRIMARY KEY,
FirstName varchar(50),
LastName varchar(50),
Email varchar(50),
Phone varchar(20)
);
This statement creates a table called Customers with five columns: CustomerID, FirstName, LastName, Email, and Phone. The CustomerID column is the primary key, which means it uniquely identifies each row in the table.
In MySQL, you can use the following statement to create the same table:
CREATE TABLE Customers (
CustomerID int PRIMARY KEY,
FirstName varchar(50),
LastName varchar(50),
Email varchar(50),
Phone varchar(20)
);
In PostgreSQL, you can use the following statement:
CREATE TABLE Customers (
CustomerID int PRIMARY KEY,
FirstName varchar(50),
LastName varchar(50),
Email varchar(50),
Phone varchar(20)
);
In SQLite, you can use the following statement:
CREATE TABLE Customers (
CustomerID INTEGER PRIMARY KEY,
FirstName TEXT,
LastName TEXT,
Email TEXT,
Phone TEXT
);
Once you have created the table, you can start inserting data into it using SQL statements.
Performing Basic SQL Operations
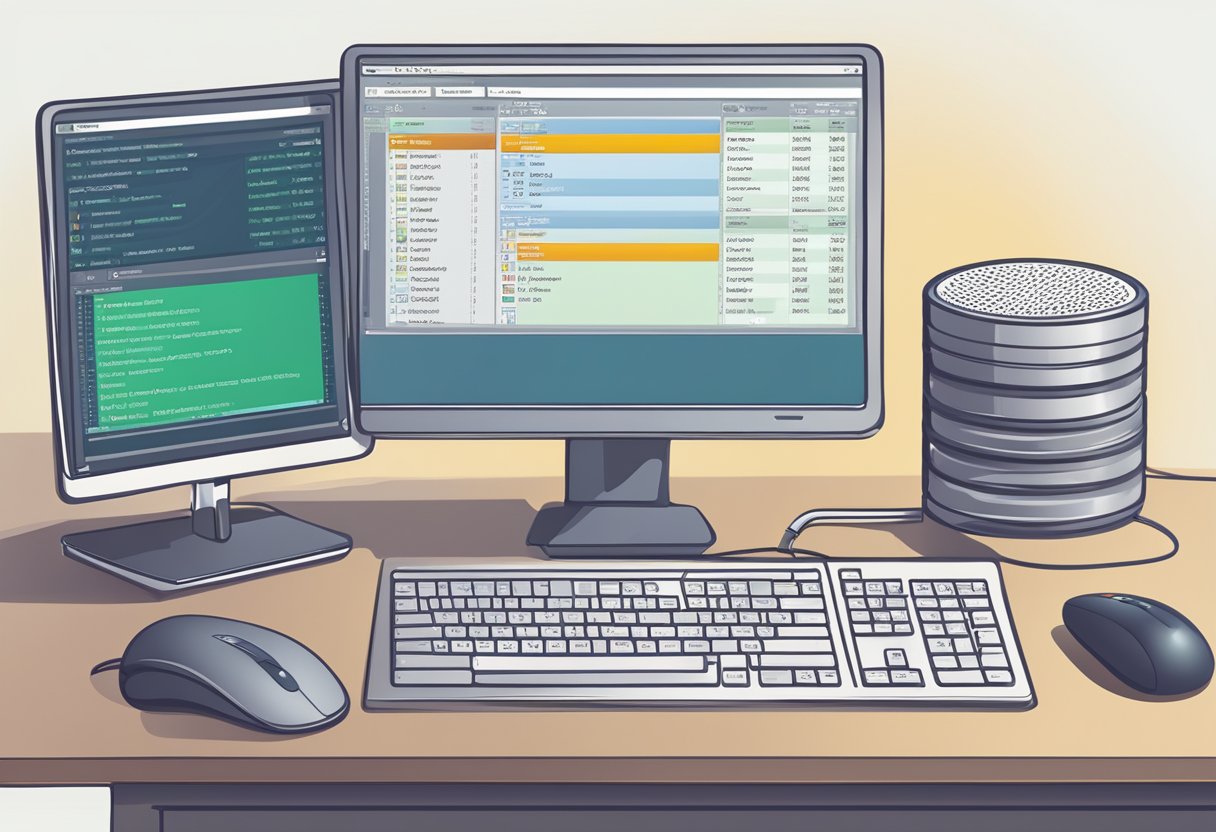
CRUD Operations: Create, Read, Update, Delete
SQL is a powerful tool for managing databases and performing CRUD operations. CRUD stands for Create, Read, Update, and Delete, which are the basic operations for managing data in a database.
To create a new record in a database, you use the CREATE statement. This statement allows you to specify the table name, column names, and values for the new record. The READ statement is used to retrieve data from the database. You can use the SELECT statement to retrieve specific columns or all columns from a table. The UPDATE statement is used to modify existing records in the database. You can use the SET clause to specify the new values for the columns. Finally, the DELETE statement is used to remove records from the database.
Writing Basic Select Statements
One of the most common SQL operations is retrieving data from a database. The SELECT statement is used to retrieve data from one or more tables in a database. You can specify the columns you want to retrieve using the SELECT clause. You can also use the WHERE clause to filter the results based on specific conditions. For example, you can retrieve all records where the value in the “name” column equals “John”.
Inserting, Updating, and Deleting Data
In addition to retrieving data, SQL also allows you to insert, update, and delete data from a database. The INSERT statement is used to add new records to a table. You can specify the column names and values for the new record. The UPDATE statement is used to modify existing records in a table. You can use the SET clause to specify the new values for the columns. The DELETE statement is used to remove records from a table.
When inserting, updating, or deleting data, it is important to specify the correct rows and columns. You can specify the rows using the WHERE clause. You can specify the columns using the SET clause for updates and the INSERT INTO clause for inserts.
Overall, SQL is a powerful tool for managing databases and performing basic operations. By understanding CRUD operations, writing basic select statements, and inserting, updating, and deleting data, you can effectively manage your database and retrieve the information you need.
Advanced SQL Query Techniques
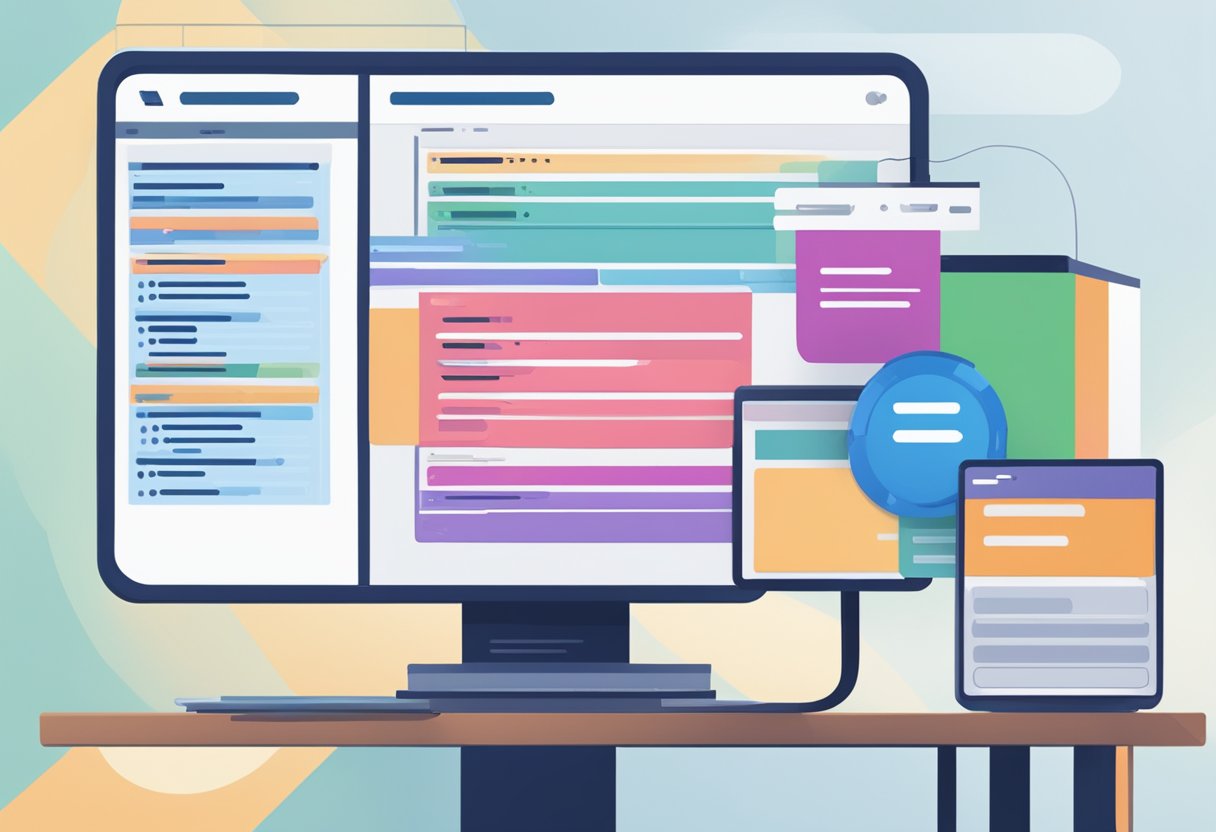
SQL is a powerful tool for managing and querying databases, and mastering advanced SQL query techniques can help you extract even more value from your data. In this section, we’ll cover some of the most important advanced SQL query techniques, including utilizing joins and subqueries, mastering the WHERE clause and conditional logic, and aggregating data with GROUP BY and HAVING.
Utilizing Joins and Subqueries
Joins and subqueries are two of the most important tools in the SQL query writer’s toolbox. Joins allow you to combine data from multiple tables based on common columns, while subqueries allow you to nest queries inside other queries to perform more complex operations.
There are several types of joins, including INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN. Each type of join has its own specific use case, and knowing when to use each type of join is an important skill for advanced SQL query writing.
Subqueries, on the other hand, allow you to perform more complex operations by nesting one query inside another. For example, you can use a subquery to filter the results of a query based on a condition that is calculated in another query.
Mastering the WHERE Clause and Conditional Logic
The WHERE clause is one of the most important parts of an SQL query, as it allows you to filter the results of a query based on specific conditions. The WHERE clause can be used with a variety of operators, including AND, OR, and BETWEEN, to create complex conditional statements that can filter data in a variety of ways.
Mastering the WHERE clause and conditional logic is an important skill for advanced SQL query writing, as it allows you to create more complex queries that can extract even more value from your data.
Aggregating Data with GROUP BY and HAVING
Aggregating data is another important advanced SQL query technique, as it allows you to summarize and analyze large amounts of data quickly and easily. The GROUP BY clause allows you to group data based on one or more columns, while the HAVING clause allows you to filter the results of a GROUP BY query based on specific conditions.
Using GROUP BY and HAVING together can allow you to perform complex data analysis tasks, such as calculating averages, sums, and other statistical measures for large datasets.
Overall, mastering advanced SQL query techniques is an important skill for anyone working with databases. By utilizing joins and subqueries, mastering the WHERE clause and conditional logic, and aggregating data with GROUP BY and HAVING, you can extract even more value from your data and gain deeper insights into your business.
Optimizing SQL Queries for Performance
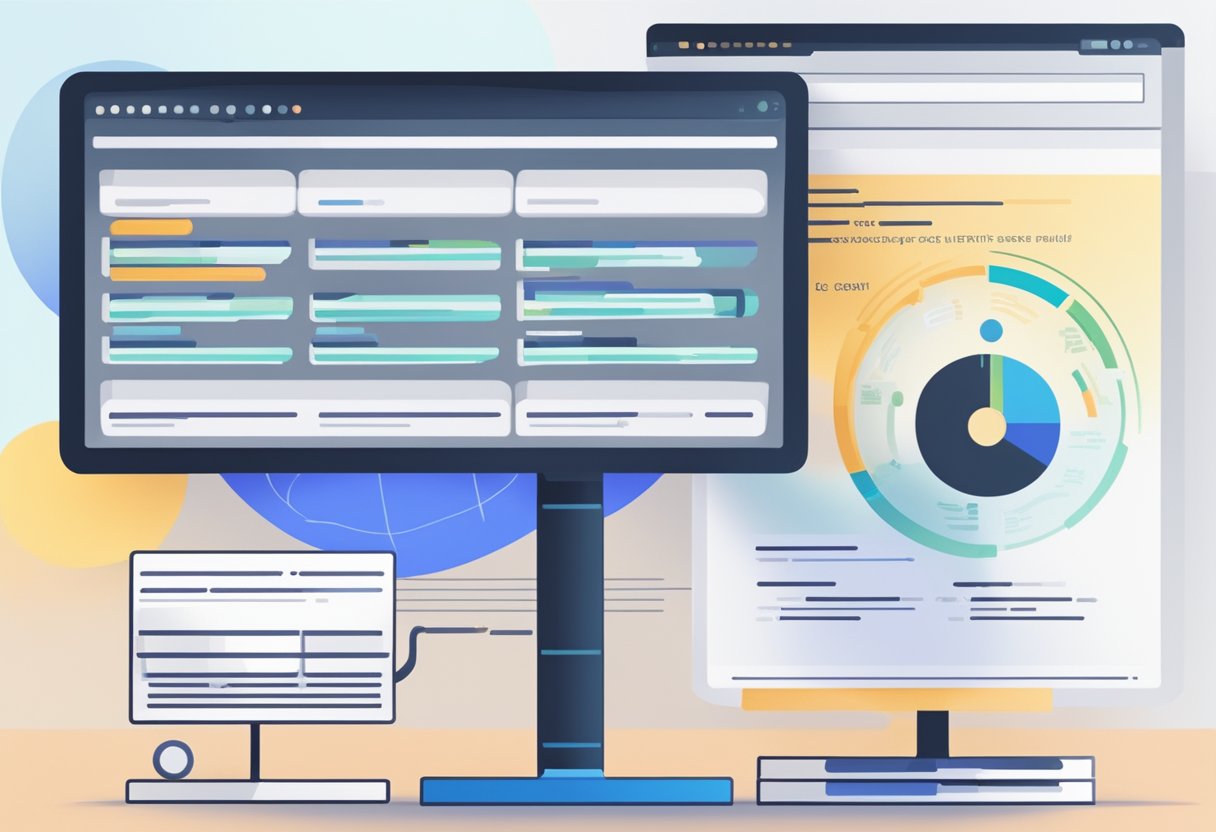
Optimizing SQL queries is a crucial aspect of database management, as poorly optimized queries can lead to slow response times, decreased efficiency, and increased resource consumption. There are several techniques that can be used to optimize SQL queries, including indexing, query planning, and analyzing.
Indexing and Query Planning
One of the most effective ways to optimize SQL queries is through indexing. Indexes are data structures that allow the database to quickly locate and retrieve data based on the values in specific columns. By creating indexes on frequently used columns, such as those used in WHERE clauses, the database can avoid full table scans and retrieve data much faster.
Another important aspect of query optimization is query planning. Query planning involves analyzing the structure of a query and determining the most efficient way to execute it. This can involve selecting the best join algorithm, choosing the most efficient index, or reordering the query to minimize the amount of data that needs to be read.
Analyzing and Tuning SQL Queries
In addition to indexing and query planning, analyzing and tuning SQL queries can also help improve performance. One technique for analyzing queries is to use the EXPLAIN statement. The EXPLAIN statement displays information about how the database will execute a query, including the order in which tables will be accessed, the join algorithms that will be used, and the indexes that will be used.
Once a query has been analyzed, it can be tuned to improve performance. This can involve rewriting the query to eliminate unnecessary joins or subqueries, or modifying the query to make better use of indexes. In some cases, it may also be necessary to adjust database settings, such as increasing the amount of memory allocated to the database or adjusting the buffer pool size.
Overall, optimizing SQL queries is essential for ensuring that database systems run efficiently and effectively. By using techniques such as indexing, query planning, and query analysis and tuning, database administrators can improve performance and minimize resource consumption.
Managing Data Across Multiple Tables
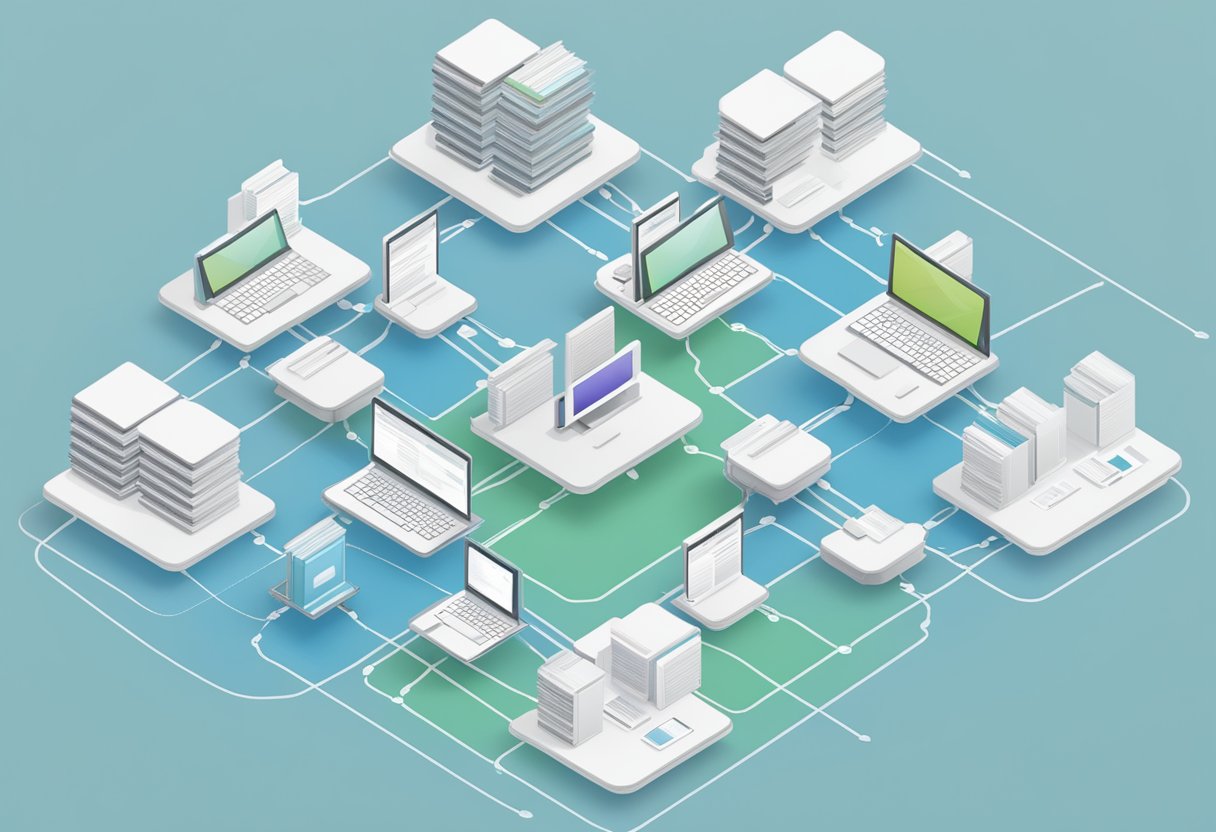
When working with databases, it is common to have data spread across multiple tables. In order to effectively manage this data, it is important to understand the relationships between the tables and how to implement set operations.
Understanding Relationships and Keys
In a relational database, tables are related to each other through keys. A key is a column or set of columns that uniquely identifies each row in a table. There are two types of keys: primary keys and foreign keys.
A primary key is a column or set of columns that uniquely identifies each row in a table. It is used to enforce data integrity and ensure that each row is unique. A foreign key is a column or set of columns that refers to the primary key of another table. It is used to establish a relationship between two tables.
Understanding the relationships between tables and the keys that define those relationships is crucial to managing data across multiple tables. By properly defining and enforcing relationships and keys, you can ensure that your data is consistent and accurate.
Implementing Set Operations
Set operations are used to combine data from multiple tables. There are three main set operations: UNION, INTERSECT, and EXCEPT.
UNION combines the results of two or more SELECT statements into a single result set. The result set contains all the rows that appear in any of the SELECT statements.
INTERSECT returns only the rows that appear in both SELECT statements.
EXCEPT returns only the rows that appear in the first SELECT statement but not in the second SELECT statement.
By using set operations, you can combine data from multiple tables in a way that makes sense for your application. For example, you might use UNION to combine data from two tables that have similar structures but contain different data.
In summary, managing data across multiple tables requires an understanding of relationships and keys, as well as the ability to implement set operations. By properly defining and enforcing relationships and using set operations to combine data, you can effectively manage data in a relational database.
Database Administration and Security
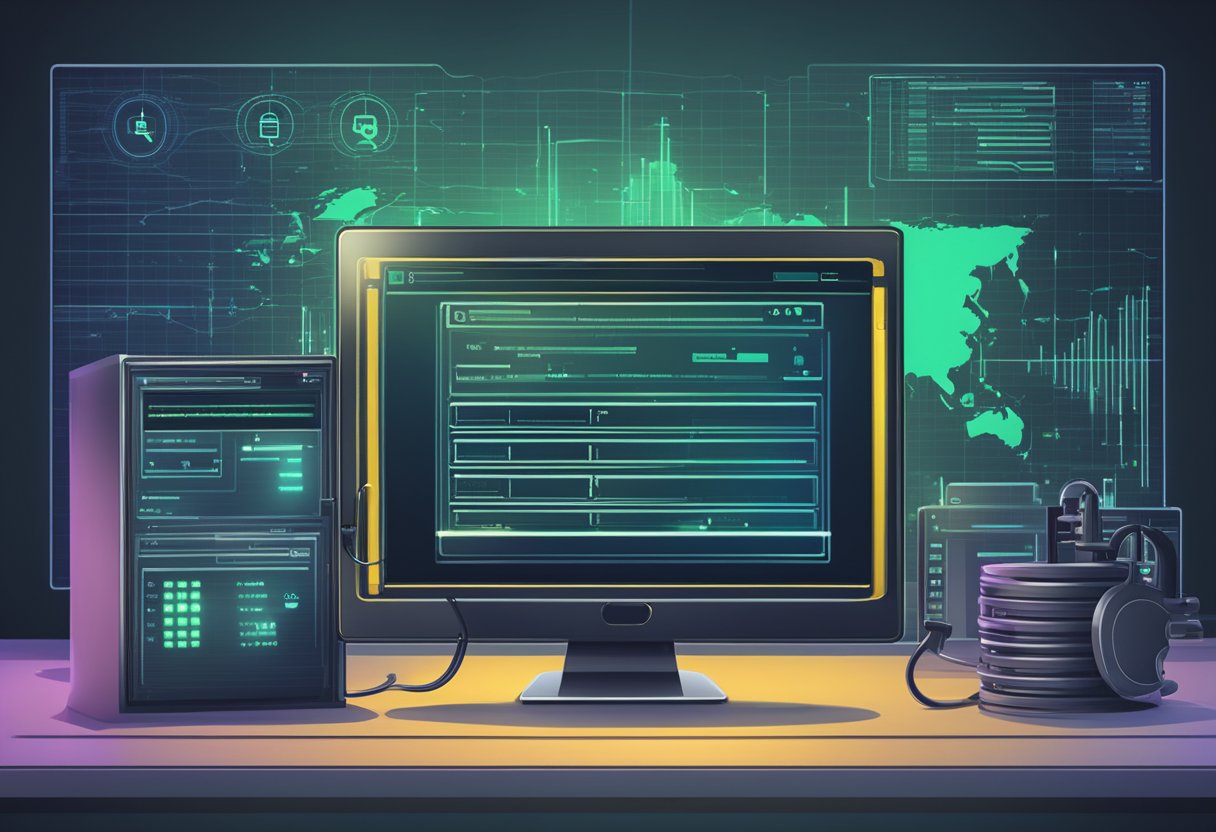
Maintaining Database Integrity
Maintaining database integrity is essential for ensuring that the data stored in the database is accurate, consistent, and reliable. This involves performing regular maintenance tasks such as backups, updates, and data validation checks. A database management system (DBMS) can help automate many of these tasks and provide tools for monitoring and managing the database.
One important aspect of maintaining database integrity is ensuring that all transactions are committed or rolled back properly. This can help prevent data corruption and ensure that the database remains in a consistent state. The COMMIT statement is used to commit a transaction, while the ROLLBACK statement is used to undo a transaction.
Another important task is managing database growth and performance. This involves monitoring the size of the database and optimizing queries to ensure that they execute efficiently. The TRUNCATE statement can be used to quickly delete large amounts of data from a table, which can help improve performance and reduce storage requirements.
Security Best Practices
Security is a critical aspect of database management, particularly for databases that contain sensitive or confidential information. There are several best practices that can help ensure the security of a database:
- Use strong passwords and implement a password policy to ensure that users choose secure passwords.
- Limit access to the database to only authorized users and roles.
- Implement encryption to protect data in transit and at rest.
- Regularly monitor and audit database activity to detect and prevent unauthorized access or activity.
- Keep the DBMS and any associated software up to date with the latest security patches and updates.
By following these best practices, database administrators can help ensure the security of their databases and the data stored within them.
Applying SQL in Different Domains
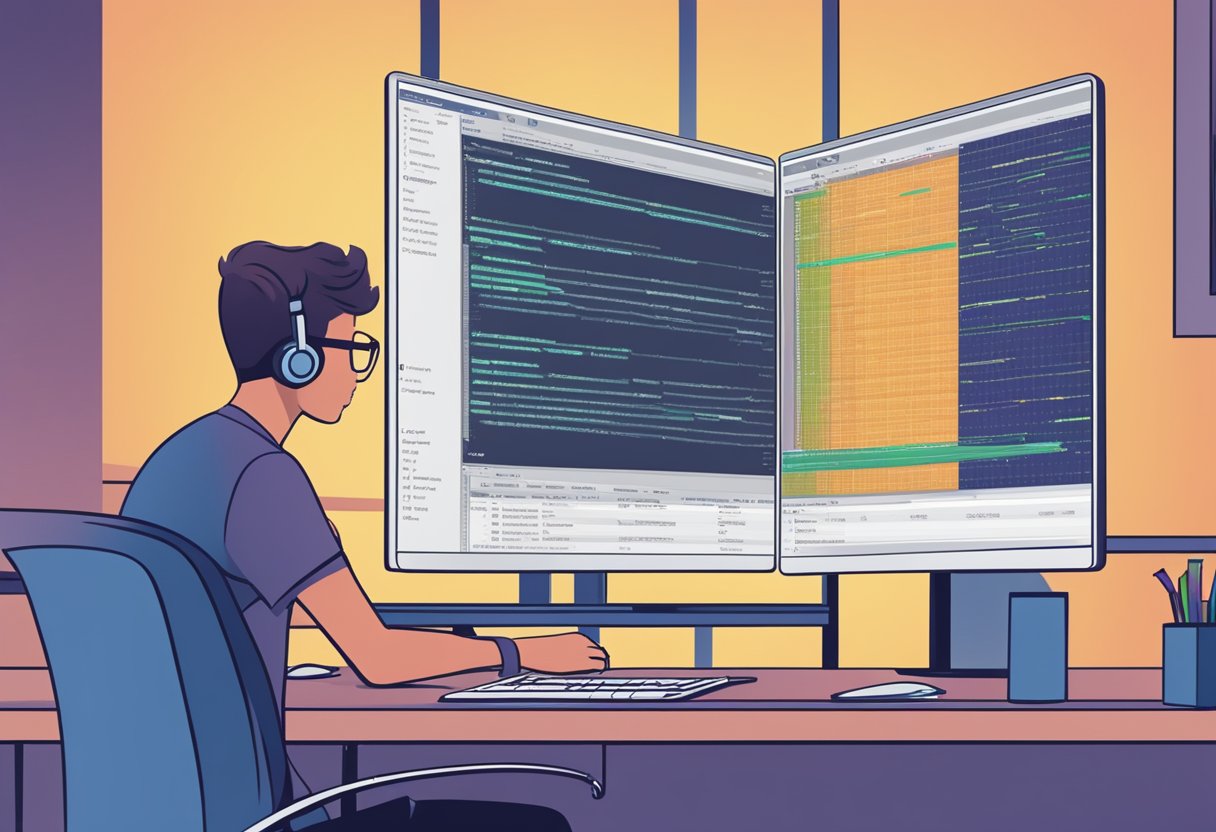
SQL is a versatile language that can be applied in various domains. Here are some examples of how SQL can be used in different fields:
SQL for Data Analysis and Reporting
SQL is widely used for data analysis and reporting. It allows users to extract and manipulate data from databases, perform calculations, and generate reports. SQL queries can be used to aggregate data, filter data, and join data from multiple tables. With SQL, users can quickly analyze large datasets and generate reports that provide insights into business performance.
SQL in Application Development
SQL is also used in application development. SQL can be used to create and manage databases, define data structures, and manipulate data. SQL is the standard language for interacting with relational databases, which are widely used in application development. SQL is used to create tables, indexes, and views, and to insert, update, and delete data. SQL is also used to write stored procedures, triggers, and functions.
SQL for Data Science and Engineering
SQL is also used in data science and engineering. SQL can be used to extract data from databases and prepare it for analysis. SQL is used to join data from multiple tables, filter data, and aggregate data. SQL can also be used to create temporary tables and views, which can be used to perform complex calculations. SQL is also used in data warehousing, where large amounts of data are stored and analyzed.
In summary, SQL is a powerful language that can be used in various domains, including data analysis and reporting, application development, and data science and engineering. SQL is a valuable skill that can help professionals in these fields to manage and manipulate data efficiently and effectively.
Learning Resources and Career Paths
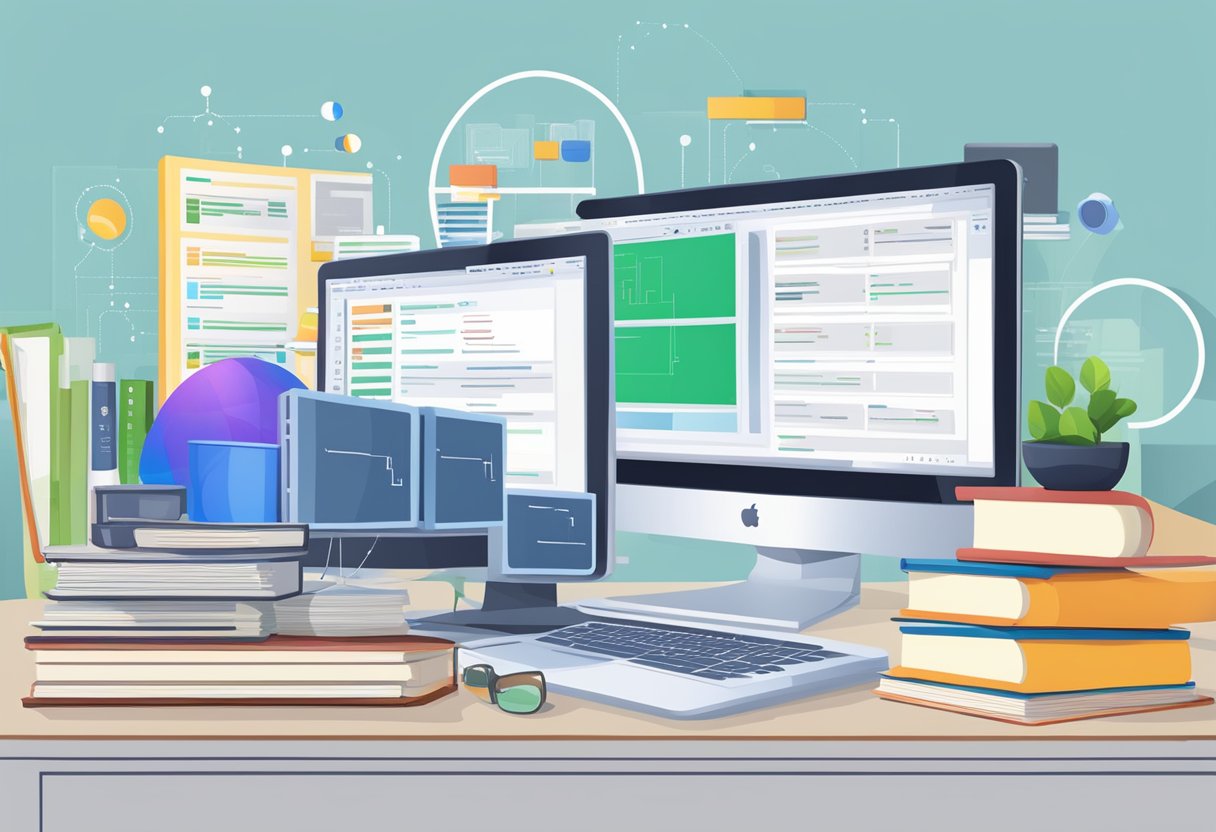
Learning SQL is essential for anyone interested in database management and queries. Fortunately, there are many resources available to help individuals learn SQL, build their portfolios, and gain experience. In this section, we will explore some of the best learning resources and career paths for SQL professionals.
Online Courses and Interactive Exercises
One of the best ways to learn SQL is through online courses and interactive exercises. LearnSQL.com is a great resource for individuals who want to learn SQL. The website offers a variety of SQL courses, including the SQL Basics Course, which teaches the basics of SQL querying, including how to retrieve data from an SQL database and build simple reports. The website also offers interactive exercises that allow individuals to practice their SQL skills.
Building a Portfolio and Gaining Experience
Building a portfolio is essential for anyone interested in a career in SQL. A portfolio should include examples of SQL queries and exercises that demonstrate an individual’s SQL skills. Individuals can gain experience by participating in SQL-related projects or by working on their own SQL projects. This will give them practical experience and help them develop their skills.
Career Opportunities for SQL Professionals
SQL professionals have many career opportunities available to them. Some of the most common career paths for SQL professionals include database administrator, data analyst, and data architect. These roles require individuals to have a strong understanding of SQL and database management. According to the US Bureau of Labor Statistics, professionals with advanced SQL skills can earn an average annual pay of $98,860, with a job growth outlook of eight percent in the coming years.
In conclusion, learning SQL is essential for anyone interested in database management and queries. There are many resources available to help individuals learn SQL, build their portfolios, and gain experience. With the right skills and experience, individuals can pursue a variety of career paths in the SQL field.